Teki
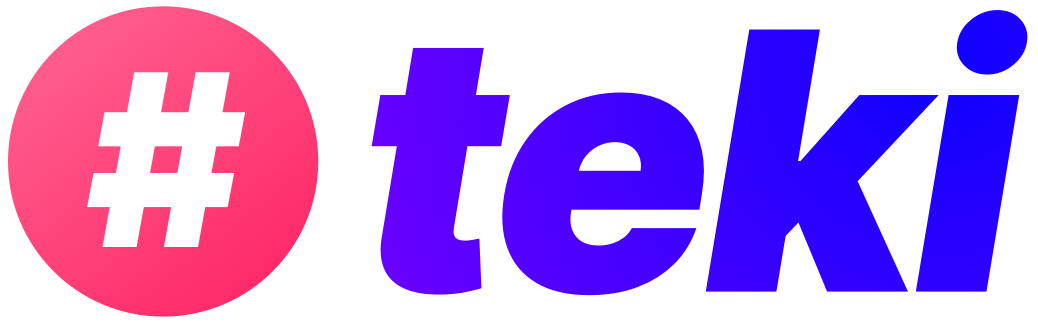
A super tiny TypeScript path parser (1.3kb gzipped!) with a surprising amount of features.
Installation
npm install --save teki
or yarn add teki
.
Usage
>> userRoute'http://localhost/user/123/messages?page=3)'
Reverse parsing
teki
can reverse parse parameter dictionaries into URLs
>> reverseUserRoute'/user/456?page=9'
Query Parameters
teki
is smart about query parameters, and will parse them
independently of order
>> queryRoute'http://localhost/myRoute?bar=hello&foo=world'
List query parameters
teki
supports list query parameters on the for ?id=1&id=2&id=3
by
using the postfixing the parameter name with *
>> listQuery'http://localhost/myRoute' >> listQuery'http://localhost/myRoute?id=1&id=2&id=3'
Optional query parameters
Query parameters can be made optional by postfixing its parameter name
with ?
>> optionalQuery'http://localhost/myRoute' >> optionalQuery''http://localhost/myRoute?foo=test')
Hash parameters
>> hashParam'http://localhost/myRoute#test'
Refining paths using regular expressions
teki
even let's you refine named parameters using regular
expressions by writing a regex after the name in angle brackets
// Only match routes where id is numeric>> userRoute'http://localhost/user/foo'null >> userRoute'http://localhost/user/123'
How does it work?
teki
achieves its small size and high performance by using
the native URL
API instead of a custom parser.
Keep in mind that this means that it will not work without a polyfill
for URL
in Internet Explorer.
API
type RouteParams
The structure of the object returned when successfully parsing a pattern.
parse
parse :: (pattern : string) => (url: string) => null | RouteParams
Parse a pattern, then accept a url to match. Returns null
on a
failed match, or a dictionary with parameters on success.
This function is curried so that its faster on repeated usages.
reverse
reverse :: (pattern : string) => (dict: RouteParams) => string
Use a dictionary to reverse-parse it back into a URL using the specified pattern.
This function will throw if the dictionary has missing parameters that are specified in the pattern.
This function is curried so that its faster on repeated usages.