Actor component of SUGOS.
SUGO-Actor works as a client of SUGO-Hub and provides modules to remote SUGO-Caller .
Table of Contents
Requirements
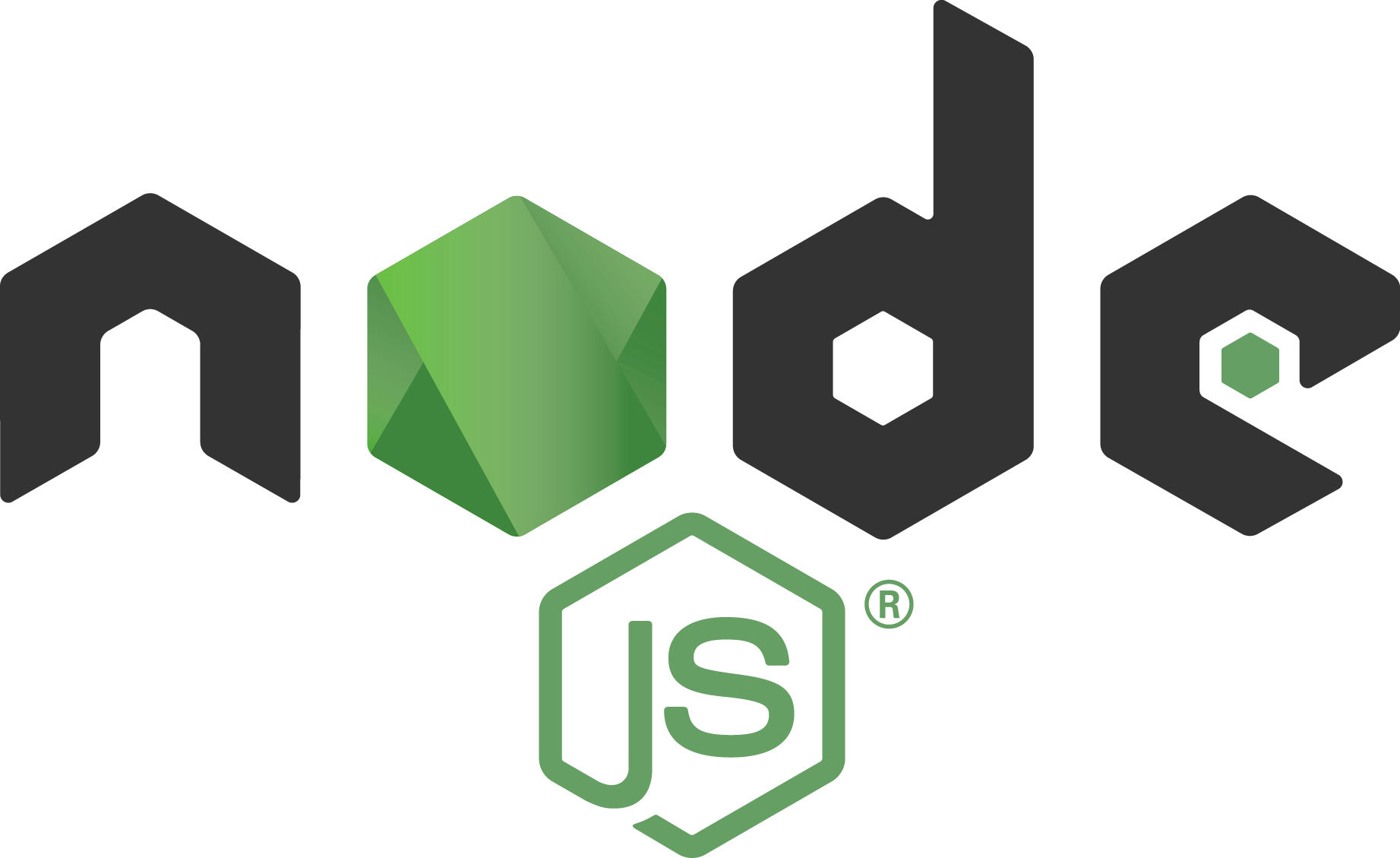

Installation
$ npm install sugo-actor --save
Usage
Create an actor instance and connect it to a [SUGO-Hub][sugo_hub_url] server.
#!/usr/bin/env node /** * This is an example to use an actor */ 'use strict' const sugoActor = const Module = sugoActor { let actor = // Connect to hub await actor}
For more detail, see API Guide
Advanced Usage
Using EventEmitter Interface
The Module class provide EventEmitter interface like .on()
, .off()
, .emit()
to communicate with remote callers.
#!/usr/bin/env node /** * This is an example for use event interface */'use strict' const sugoActor = const Module = sugoActorconst fs = { let actor = // Connect to hub await actor}
$spec
Description with You can describe a module with $spec
property.
The spec object must conform to module_spec.json, a JSON-Schema.
#!/usr/bin/env node /** * This is an example to define spec on module * * @see https://github.com/realglobe-Inc/sg-schemas/blob/master/lib/module_spec.json */'use strict' const sugoActor = const Module = sugoActorconst fs = { let actor = // Connect to hub await actor}
Declare a Single Function as Module
Sometimes you do not want multiple module methods, but only one function. Just declaring a function as module would do this.
#!/usr/bin/env node /** * This is an example to use a function module */'use strict' const sugoActor = const Module = sugoActorconst fs = { let actor = // Connect to hub await actor}
Add Auth Configuration
You can pass auth config to SUGO-Hub by setting auth
field on the constructor.
#!/usr/bin/env node /** * This is an example to use an auth * @see https://github.com/realglobe-Inc/sugo-hub#use-authentication */'use strict' const sugoActor = const Module = sugoActorconst fs = { let actor = // Connect to hub await actor}
Detect Caller Join/Leave
Actor emits CallerEvents.JOIN
and CallerEvents.LEAVE
events each time a caller connected/disconnected.
#!/usr/bin/env node /** * This is an example to detect caller join/leave */'use strict' const sugoActor = const CallerEvents = sugoActor const JOIN LEAVE = CallerEvents { let actor = actor actor // Connect to hub await actor}
License
This software is released under the Apache-2.0 License.